Try These Advanced SQL Join Techniques
Table of Contents
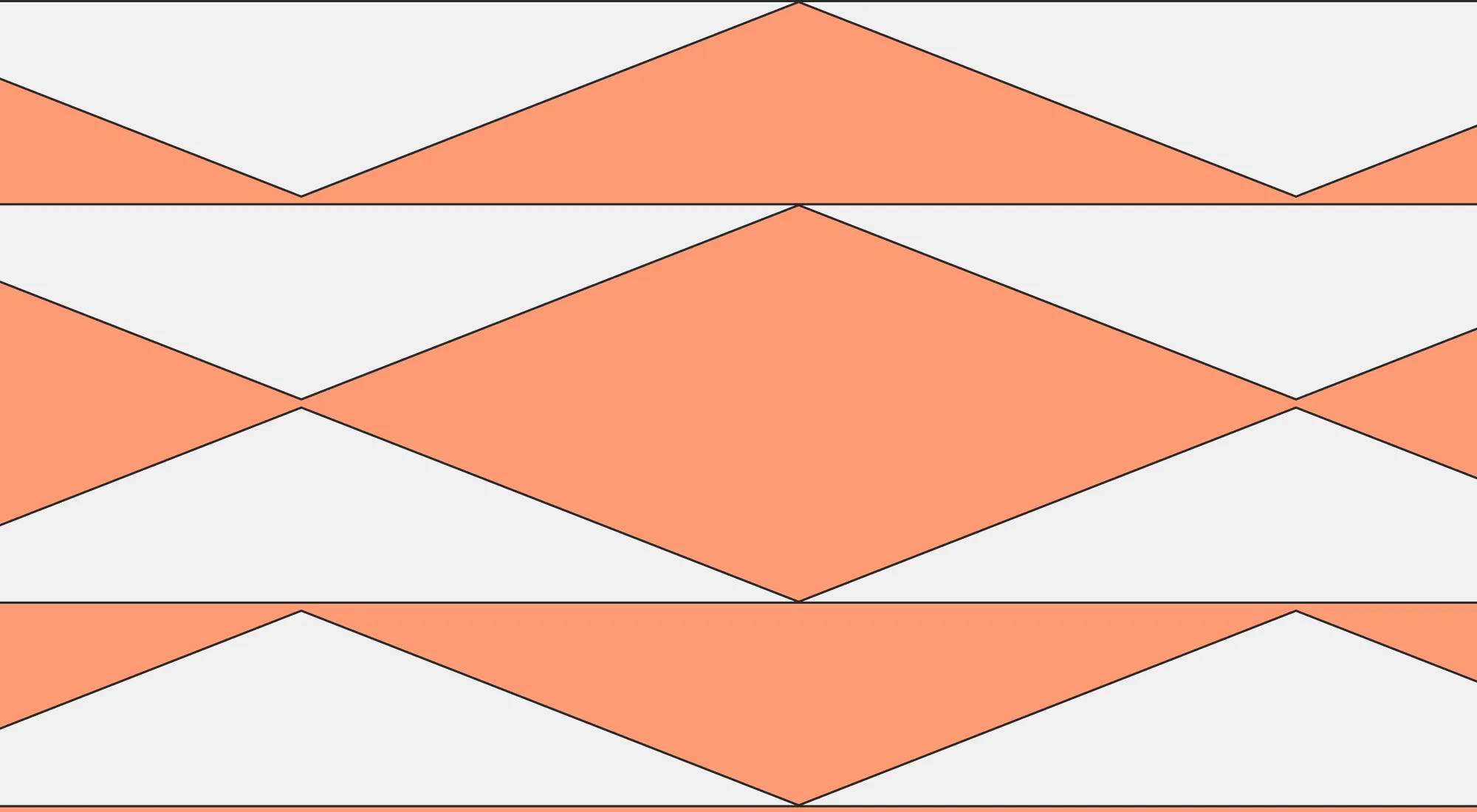
If your SQL queries aren’t giving you the desired results, it might be time to rethink your approach. Advanced SQL join techniques help you work with complex data, improve query efficiency, and avoid mistakes that lead to inaccurate analysis. Whether combining multiple tables, using subqueries for deeper analysis, or optimizing performance, effectively structuring your joins can save time and prevent costly mistakes.
SQL joins are fundamental pieces of complex data queries, but basic joins can only take you so far. As datasets grow and queries become more intricate, advanced SQL joins are the key to writing efficient, precise queries.
In this guide, we’ll explore advanced join techniques that help you write faster, more efficient queries while avoiding common pitfalls. You’ll learn how to work with multiple joins in a single query, leverage subqueries, apply complex join conditions, and optimize performance. By the end, you’ll have a solid foundation to tackle even the trickiest data relationships.
Already comfortable with the basics? This post builds on our previous SQL joins guide, diving deeper into techniques that data engineers and analysts can apply immediately. Think of this as your SQL joins playbook, packed with practical examples and real-world applications to make your queries more powerful. Let’s get started.
How to add multiple joins in a single query
When working with multiple tables, a single join often isn’t enough. Combining data from several sources in one query allows for a more comprehensive analysis, but structuring these joins correctly is crucial for readability and performance.
Syntax for multiple joins
SQL allows you to stack multiple joins within a query, but the order of execution matters. The database processes joins from left to right unless parentheses specify otherwise. Here’s an example using a scenario where we analyze customer purchases across different sales channels:
SELECT
c.customer_name,
ch.channel_name,
SUM(o.order_total) AS total_spent,
COUNT(o.order_id) AS total_orders
FROM customers c
JOIN orders o ON c.customer_id = o.customer_id
JOIN sales_channels ch ON o.channel_id = ch.channel_id
WHERE o.order_date >= '2024-01-01'
GROUP BY c.customer_name, ch.channel_name;
This query:
- Joins customer data with orders to see how much each customer has spent.
- Connects sales channels to understand where purchases are happening.
- Filters data by date to focus on recent transactions.
Order of execution and impact on performance
Each additional join increases computational complexity. Poorly structured joins can slow down query performance, especially with large datasets. To optimize multiple joins:
- Start with the most restrictive join to filter unnecessary data early.
- Use INNER JOIN when possible to exclude irrelevant records.
- Leverage indexes on commonly joined columns to improve lookup speed.
Best practices for readability and maintainability
Long SQL queries can be difficult to debug. Keep your joins clean and easy to follow:
- Use table aliases (c, o, ch) to shorten queries while maintaining clarity.
- Indent each JOIN statement to improve readability.
- Comment complex joins to explain relationships between tables.
Using subqueries with joins
Subqueries allow you to filter and aggregate data dynamically within a query, making them a flexible alternative to standard joins. While joins combine datasets directly, subqueries pre-process data before merging results, which can improve efficiency and readability. Knowing when to use subqueries instead of joins can help streamline complex queries and prevent unnecessary calculations.
Subqueries vs. joins: when to use each
Joins and subqueries both retrieve related data, but they serve different purposes. Joins are best when combining multiple tables and returning several columns from each. Conversely, subqueries are useful for filtering, aggregating, or ranking data before merging it with the main query. If performance is a concern, testing both approaches can help determine which is more efficient.
For example, an e-commerce company analyzing top-selling products may want to identify items that generated more than $50,000 in revenue. Instead of using a join, a subquery inside a WHERE clause can pre-calculate total revenue before filtering:
SELECT product_name, total_revenue
FROM (
SELECT p.product_name, SUM(o.quantity * o.unit_price) AS total_revenue
FROM products p
JOIN orders o ON p.product_id = o.product_id
GROUP BY p.product_name
) product_totals
WHERE total_revenue > 50000;
This approach calculates revenue per product first, simplifying the main query and improving readability. Since the filtering happens after aggregation, the query avoids unnecessary calculations on lower-selling products.
Nested queries for filtering and aggregation
Subqueries can also be used inside SELECT statements to calculate values dynamically without affecting the main dataset. Suppose a subscription service wants to find each user's most recent payment date. A nested query inside the SELECT statement can return the latest transaction for each user:
SELECT u.user_name,
(SELECT MAX(p.payment_date)
FROM payments p
WHERE p.user_id = u.user_id) AS last_payment_date
FROM users u;
Instead of performing a full join, this approach retrieves only the most recent payment per user, reducing the number of records scanned and simplifying query logic.
Performance trade-offs
While subqueries add flexibility, they are not always the most efficient option. If a subquery returns a large dataset, it can slow down performance, making joins or common table expressions (CTEs) a better choice. Indexing filtered columns can help improve subquery speed, ensuring queries remain responsive even with large datasets. Breaking complex queries into smaller steps also makes them easier to debug and optimize.
Understanding when to use subqueries and how to structure them effectively ensures that queries remain efficient, readable, and scalable as data grows.
When and how to use complex join conditions
Basic joins work well when tables have simple one-to-one or one-to-many relationships, but real-world data often requires more precise filtering and matching. Complex join conditions help analysts merge data accurately by incorporating multiple criteria, handling computed values, and working with composite keys. These techniques allow for more nuanced insights while ensuring queries remain efficient.
A single join condition isn’t always enough when dealing with datasets that require specific filtering criteria. Consider an e-commerce company tracking high-value repeat customers, where they need to analyze customers who made a first purchase of at least $500 and returned within the last 90 days. A standard join wouldn't capture both conditions, but a subquery with multiple conditions can refine the results:
SELECT c.customer_id, c.customer_name, r.first_order_date, r.latest_order_date, r.total_spent
FROM customers c
JOIN (
SELECT customer_id,
MIN(order_date) AS first_order_date,
MAX(order_date) AS latest_order_date,
SUM(order_total) AS total_spent
FROM orders
GROUP BY customer_id
HAVING MIN(order_total) >= 500 AND MAX(order_date) >= NOW() - INTERVAL 90 DAY
) r ON c.customer_id = r.customer_id;
This query filters customers before joining them to the main dataset, ensuring only high-value repeat buyers are included. This approach reduces query complexity and improves performance by pre-aggregating order totals and filtering early.
Sometimes, data in separate tables doesn’t align perfectly, requiring computed joins to match values dynamically. A subscription-based business might need to link users to the correct billing cycle based on the closest invoice date before their subscription start date. Since standard joins can’t handle this scenario directly, a subquery helps identify the most relevant invoice:
SELECT s.user_id, u.user_name, s.current_tier, t.previous_tier
FROM subscriptions s
JOIN (
SELECT user_id, subscription_tier AS previous_tier
FROM subscription_history
WHERE change_date = (
SELECT MAX(change_date)
FROM subscription_history
WHERE user_id = s.user_id
)
) t ON s.user_id = t.user_id;
This ensures each user is matched to their most recent prior subscription before any tier changes. Without this logic, a simple join could return outdated or irrelevant records, affecting billing and user tracking.
In some datasets, a single column isn’t enough to define relationships between tables. Composite keys, where two or more columns uniquely identify a record, require joins on multiple fields to maintain accuracy. A healthcare provider tracking patient visits and lab results needs to ensure each test is linked to the correct appointment by both patient ID and visit date:
SELECT v.visit_id, p.patient_name, l.lab_test, l.result
FROM visits v
JOIN patients p ON v.patient_id = p.patient_id
JOIN lab_results l
ON v.patient_id = l.patient_id
AND v.visit_date = l.test_date;
By joining on both patient_id and visit_date, this query prevents incorrect test assignments while ensuring accurate reporting. Without these conditions, lab results could be mismatched, leading to incorrect medical records.
Complex joins can strain performance, but optimizing query structure helps avoid bottlenecks. Indexing multiple columns used in conditions speeds up lookups, while using INNER JOINs where possible reduces unnecessary rows early in execution.
Running an execution plan (EXPLAIN ANALYZE) helps identify inefficient operations and adjust query structure accordingly. Breaking queries into temporary tables or common table expressions (CTEs) improves readability and debugging for highly complex joins.
Using these techniques ensures SQL queries remain efficient and maintainable, even as datasets grow in complexity.
How to optimize SQL join performance
Optimizing SQL joins is essential for improving query efficiency and ensuring databases run smoothly, especially when working with large datasets. Poorly structured joins can slow queries, increase processing costs, and create unnecessary complexity.
One of the most effective ways to optimize joins is by using indexes on frequently joined columns. Indexing helps reduce the number of records scanned during execution, significantly improving lookup speed. However, excessive indexing can slow inserts and updates, so it's important to strike a balance by prioritizing the most frequently queried fields.
Another critical optimization strategy is avoiding redundant joins. Unnecessary joins increase query complexity and execution time, particularly in analytics dashboards or reporting queries. Filtering data before performing joins, aggregating results in pre-processed tables, or using subqueries to limit dataset size can lead to more efficient query execution.
Additionally, analyzing query execution plans helps identify slow-performing joins by revealing full table scans, inefficient index usage, or costly sorting operations. Running an EXPLAIN ANALYZE on queries allows analysts to pinpoint bottlenecks and restructure joins accordingly.
By reducing unnecessary joins, indexing key columns, and leveraging execution plans, SQL users can significantly improve query performance while keeping accurate and efficient data retrieval.
5 common mistakes in SQL joins and how to avoid them
SQL joins are fundamental to working with relational databases, but small mistakes can lead to incorrect results, performance issues, or data integrity problems. Whether analyzing customer transactions, tracking product inventory, or generating financial reports, ensuring joins are structured correctly is essential for accurate and efficient queries.
Incorrect join conditions
One of the most common mistakes is misconfiguring join conditions, which can cause missing data, duplicate records, or incorrect calculations. Suppose an online streaming service analyzes user activity and wants to track what content each subscriber has watched. A poorly written join could cause incorrect matching between users and their watch history:
SELECT u.user_name, v.video_title, v.genre
FROM users u
JOIN watch_history w ON u.user_id = w.account_id
JOIN videos v ON w.video_id = v.video_id;
Here, the JOIN mistakenly matches user_id from users with account_id in watch_history, which could result in missing data or incorrect mappings. The correct query should ensure the join condition matches the correct user identifier:
SELECT u.user_name, v.video_title, v.genre
FROM users u
JOIN watch_history w ON u.user_id = w.user_id
JOIN videos v ON w.video_id = v.video_id;
Analysts can prevent data mismatches and ensure queries return accurate results by carefully verifying join conditions.
Missing NULL handling
Failing to account for NULL values can lead to misleading reports, particularly when using INNER JOIN, which excludes rows without a match. Suppose a subscription-based e-learning platform wants to list all users and their latest completed course. If a student hasn’t finished any courses yet, they will be omitted from the results:
SELECT u.user_name, c.course_name, c.completion_date
FROM users u
INNER JOIN course_completions c ON u.user_id = c.user_id;
To include all users, even those who haven’t completed a course, a LEFT JOIN should be used instead:
SELECT u.user_name, COALESCE(c.course_name, 'No course completed') AS last_course,
COALESCE(c.completion_date, 'N/A') AS completion_date
FROM users u
LEFT JOIN (
SELECT user_id, course_name, MAX(completion_date) AS completion_date
FROM course_completions
GROUP BY user_id, course_name
) c ON u.user_id = c.user_id;
This approach ensures the query includes all users while substituting NULL values with meaningful placeholders.
Wrong join type selection
Choosing the wrong join type can distort data analysis. Suppose a logistics company needs to track all scheduled deliveries, including those that haven’t been assigned a driver yet. Using an INNER JOIN would exclude unassigned deliveries:
SELECT d.delivery_id, d.package_id, dr.driver_name
FROM deliveries d
INNER JOIN drivers dr ON d.driver_id = dr.driver_id;
Since some deliveries may not have a driver assigned, a LEFT JOIN should be used instead:
SELECT d.delivery_id, d.package_id, COALESCE(dr.driver_name, 'Not assigned') AS driver
FROM deliveries d
LEFT JOIN drivers dr ON d.driver_id = dr.driver_id;
Now, all deliveries remain in the results, and any unassigned deliveries are clearly marked.
Unnecessary joins
Adding unnecessary joins increases query complexity and slows down performance. Consider a music streaming service that wants to calculate the total playtime per artist. A poorly optimized query might introduce unnecessary joins that slow down execution:
SELECT a.artist_name, u.user_name, SUM(s.song_duration) AS total_playtime
FROM artists a
JOIN albums al ON a.artist_id = al.artist_id
JOIN songs s ON al.album_id = s.album_id
JOIN play_history p ON s.song_id = p.song_id
JOIN users u ON p.user_id = u.user_id
GROUP BY a.artist_name, u.user_name;
If the goal is to analyze playtime per artist, there’s no need to join users. A more efficient query removes unnecessary joins and improves readability:
SELECT a.artist_name, SUM(s.song_duration) AS total_playtime
FROM artists a
JOIN albums al ON a.artist_id = al.artist_id
JOIN songs s ON al.album_id = s.album_id
JOIN play_history p ON s.song_id = p.song_id
GROUP BY a.artist_name;
Reducing the number of joins speeds up execution while still capturing the essential data.
Ensuring data integrity
Poorly implemented joins can cause duplicate records, missing data, or inconsistencies in reporting. Suppose a ride-sharing platform is analyzing driver payouts and wants to ensure that payments are calculated correctly. An incorrect join might result in inflated earnings due to multiple records per ride:
SELECT d.driver_name, SUM(p.amount_paid) AS total_earnings
FROM drivers d
JOIN rides r ON d.driver_id = r.driver_id
JOIN payments p ON r.ride_id = p.ride_id
GROUP BY d.driver_name;
Total earnings could be overstated if the payments table contains multiple transactions per ride (e.g., a base fare and an incentive bonus). The correct approach is to pre-aggregate payments before joining:
SELECT d.driver_name, SUM(p.total_paid) AS total_earnings
FROM drivers d
JOIN (
SELECT ride_id, SUM(amount_paid) AS total_paid
FROM payments
GROUP BY ride_id
) p ON d.driver_id = p.driver_id
GROUP BY d.driver_name;
This method ensures accurate payout calculations while maintaining query efficiency.
How to troubleshoot SQL join queries
When a SQL join isn’t returning the expected results, the first step is determining whether the issue lies in the join condition, filters, or data structure. Missing records often indicate that an INNER JOIN is unintentionally excluding unmatched rows, which can be resolved by switching to a LEFT JOIN when appropriate.
Duplicate rows may stem from one-to-many relationships, where multiple matches in a joined table cause unintended repetition. In such cases, aggregating data before joining or applying DISTINCT can help ensure cleaner results. Running COUNT(*) before and after a join can also highlight whether records are filtered out unintentionally or duplicated unexpectedly.
If performance is the issue, using EXPLAIN ANALYZE helps identify bottlenecks caused by full table scans, missing indexes, or inefficient join orders. Queries that take too long to execute often benefit from indexing frequently used join columns, filtering data before joining, or restructuring joins to eliminate redundant operations.
Breaking a query into smaller test cases can also reveal where errors arise before applying changes to the entire dataset. Debugging SQL joins is about fixing errors and refining queries to ensure accuracy, efficiency, and long-term maintainability.
Advanced SQL joins in action
Mastering advanced SQL joins allows analysts and engineers to write efficient, accurate, and scalable queries. Using the right join types, optimizing performance, and troubleshooting effectively ensures data remains reliable and queries run smoothly. By applying these techniques, SQL users can confidently handle complex data relationships and extract deeper insights for better decision-making.
Advanced SQL joins: FAQs
What is the best way to debug a slow join query?
The most effective approach is to analyze the execution plan using EXPLAIN ANALYZE, which highlights full table scans, missing indexes, and inefficient join orders.
Optimizing performance may involve indexing frequently joined columns, filtering datasets before joining, or restructuring queries to eliminate unnecessary operations. If a query remains slow after indexing, consider breaking it into smaller, more manageable parts to isolate performance bottlenecks.
How do I decide between using a join or a subquery?
Joins are ideal for combining related tables and retrieving multiple columns from each, while subqueries are better suited for filtering, aggregations, or ranking data before merging it into the main query.
Testing both approaches can help determine the most efficient solution when performance is a concern.
What is the most efficient way to handle NULL values in joins?
Using LEFT JOIN instead of INNER JOIN ensures unmatched rows are retained when necessary, while functions like COALESCE or IFNULL replace NULL values with defaults to prevent missing data in reports.
If NULLs affect joins, checking for missing relationships in the data source can help resolve inconsistencies.
Can too many joins slow down my query?
Yes, excessive joins can increase query complexity and execution time, especially with large datasets. Optimizing queries by removing unnecessary joins and indexing key columns can significantly improve speed. Running EXPLAIN ANALYZE helps identify which joins are slowing execution.
What should I do if my join is returning duplicate records?
Duplicate records often result from one-to-many relationships with multiple matches in the joined table. To prevent duplication, check the granularity of the data, use DISTINCT where needed, or apply aggregation functions like GROUP BY to consolidate results.
Testing queries with a smaller dataset can also help identify the source of duplication. Additionally, reviewing the join condition for unintended cross-joins can help eliminate excess rows.